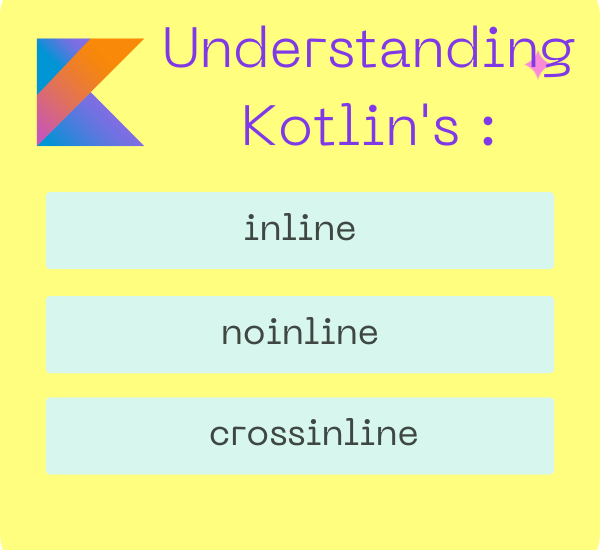
An inline function in Kotlin is a function whose body is copied directly into the calling code at compile time, rather than being called through the usual function call mechanism. By eliminating the function call overhead, Kotlin optimizes the performance of small and frequently called functions.
Here is a simple example :
inline fun greet(name: String): String {
return "Hello, $name!"
}
fun main() {
val greeting = greet("Alice")
println(greeting) // Output: Hello, Alice!
}
In the example above, the greet
function is marked with the inline
keyword. Instead of calling the function normally, Kotlin inserts the code directly into the call site during compilation. As a result, there’s no function call overhead, making it efficient.
Why Use Inline Functions?
Inline functions offer several benefits, particularly in performance-sensitive scenarios:
- Eliminates Function Call Overhead: By replacing function calls with the actual code, inline functions reduce the overhead of calling and returning from functions.
- Improves Performance: Particularly useful for small, frequently called functions, such as simple utilities or lambda-based operations.
- Lambda Optimizations: When used with higher-order functions, inline functions help optimize lambda expressions, preventing unnecessary object creation.
Inline Functions with Higher-Order Functions
Kotlin’s inline functions are especially valuable when working with higher-order functions (functions that take other functions as parameters). Let’s look at an example:
inline fun calculate(operation: (Int, Int) -> Int): Int {
val x = 5
val y = 3
return operation(x, y)
}
fun main() {
val result = calculate { a, b -> a + b }
println(result) // Output: 8
}
When not to Use Inline Functions
Although inline functions provide performance benefits, they are not always appropriate. Here are some situations where you should avoid them:
- Large Function Bodies: If the function body is too large, inlining can increase the code size, which may negatively impact performance.
- Recursive Functions: Inline functions cannot be recursive, as inlining a recursive function would result in infinite expansion.
- Complex Logic: If the function includes complex operations or multiple paths (e.g., loops, conditional statements), inlining may not yield significant benefits.
Why use noinline?
The noinline
keyword prevents a specific lambda from being inlined. This is useful when you want to pass the lambda further, store it, or execute it in another context without it being inlined.
inline fun processNumbers(
x: Int,
y: Int,
noinline operation: (Int, Int) -> Int
): Int {
println("Before operation")
val storedOperation = operation(x, y) // Storing the lambda, so it can't be inlined
println("After operation")
return storedOperation
}
val result = processNumbers(4, 2) { a, b -> a * b }
println("Result: $result")
// Output :
Before operation
After operation
Result: 8
In this example:
- The
transform
lambda is marked asnoinline
, so it won’t be inlined. - You can pass this lambda to another function or store it in a variable later in the code.
Why use crossinline?
The crossinline
keyword ensures that a lambda cannot return from the enclosing function. This is particularly important when the lambda is used in a situation where returning directly from the lambda would break the flow (like when starting a new thread).
fun main() {
myFunction(
block = {
println("Normal block, non-local return allowed")
return
},
anotherBlock = {
println("Crossinline block, non-local return not allowed")
//return // would cause an error here
}
)
}
inline fun myFunction(block: () -> Unit, crossinline anotherBlock: () -> Unit) {
block() // This lambda can do a non-local return
anotherBlock() // This lambda cannot do a non-local return
}
Type | Description | When to Use |
---|---|---|
inline | The function's body is copied directly into the calling site. This reduces the overhead of function calls and improves performance. | Use when you want to optimize performance for small, frequently called functions or higher-order functions with lambdas. |
noinline | Prevents a specific lambda parameter from being inlined. This allows the lambda to be passed further or stored in a variable. | Use when you need to pass the lambda to another function or store it as a variable, making inlining inappropriate. |
crossinline | Prevents the lambda from using the `return` keyword to exit the outer function, ensuring the lambda stays within its own context. | Use when the lambda is executed in another context (e.g., a thread or coroutine) and you want to prevent early exits from the outer function. |
Conclusion
Inline functions are a powerful feature in Kotlin that help reduce overhead, optimize performance, and make higher-order functions more efficient. However, they are best used for small, simple functions or frequently called utilities to maximize their benefits.
Remember to use noinline
when you need to prevent specific lambdas from being inlined and crossinline
when you want to ensure lambdas don’t disrupt the flow of your function. Experiment with inline functions in your code to see the performance improvements for yourself, and remember to use them wisely to strike the right balance between performance and code maintainability.