Dialogs are essential in apps for showing progress or getting user attention. In this guide, we’ll create a simple loading dialog in Jetpack Compose with a CircularProgressIndicator
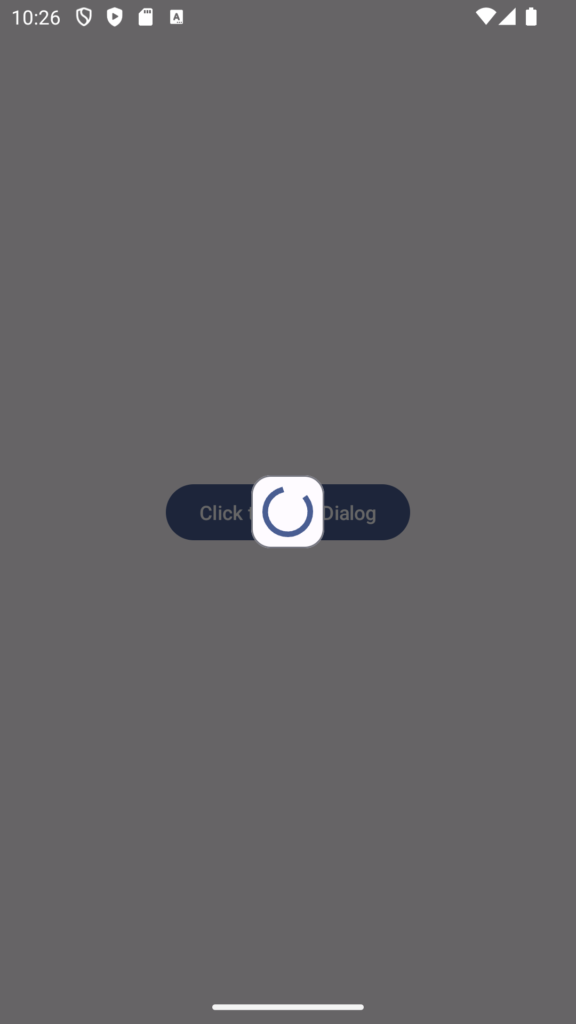
@Composable
fun LoadingDialog() {
var showDialog by remember { mutableStateOf(false) }
Box(
contentAlignment = Alignment.Center,
modifier = Modifier.fillMaxSize()
) {
Button(onClick = { showDialog = true }) {
Text(text = "Click to show Dialog")
}
}
if (showDialog) {
Dialog(
onDismissRequest = { showDialog = false },
properties = DialogProperties(usePlatformDefaultWidth = false)
) {
Box(modifier = Modifier.padding(8.dp), contentAlignment = Alignment.Center) {
Surface(
shape = RoundedCornerShape(14.dp),
border = BorderStroke(1.dp, MaterialTheme.colorScheme.outline),
color = MaterialTheme.colorScheme.surface
) {
CircularProgressIndicator(
modifier = Modifier.padding(8.dp).size(36.dp)
)
}
}
}
}
}
Key Points
- State Management: Use
remember
to manage the visibility of the dialog. - Dialog Design: Customize with
Dialog
andSurface
for rounded shapes and styling. - Loader: Add a
CircularProgressIndicator
inside the dialog to show the loading state.
How It Works
- A button toggles the visibility of the dialog.
- The dialog contains a rounded
Surface
and a loader in the center. - The
onDismissRequest
ensures the dialog can be dismissed by clicking outside or pressing back.
Usage
Call LoadingDialog()
in your setContent
function to test:
setContent {
MaterialTheme {
LoadingDialog()
}
}